methode
rekursif adalah perulangan dengan menggunakan teknik memanggil methode
itu sendiri. reskursif tidak mengunakan teknik perulangan iteratif
(misalnya for atau while). berikut contoh programnya:SORTING – NON GUI
Nama program: bubbleSort.java
Output program:
7 5 3 8 2
2 3 5 7 8
Nama program: SelectionSort.java
Output program:
9 69 96 97 47
9 47 69 96 97
Nama program: InsertionSort.java
Output program:
7 5 3 8 2
2 3 5 7 8
SORTING – GUI
Nama program: SortingGui.java
Nama program: ProsesSorting
Output program:
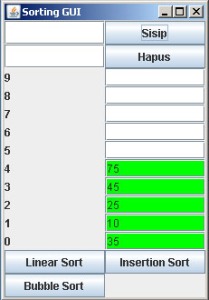
Elemen array sebelum diurutkan
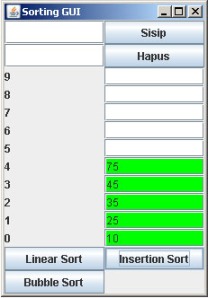
Elemen array setelah diurutkan
public class BubbleSort { |
public static void main(String[] args) { |
int array[] = new int [ 5 ]; |
array[ 0 ] = 7 ; |
array[ 1 ] = 5 ; |
array[ 2 ] = 3 ; |
array[ 3 ] = 8 ; |
array[ 4 ] = 2 ; |
for ( int index= 0 ; index<array.length; index++) { |
System.out.print(array[index] + ” “); |
} |
System.out.println(“”);<!--more--> |
int kanan; |
int kiri; |
for (kanan=array.length- 1 ; kanan> 0 ; kanan–) { |
for (kiri= 0 ; kiri<kanan; kiri++) { |
if ( array[kiri] > array[kiri+ 1 ] ) { |
int temp = array[kiri]; |
array[kiri] = array[kiri+ 1 ]; |
array[kiri+ 1 ] = temp; |
} |
} |
} |
7 5 3 8 2
2 3 5 7 8
Nama program: SelectionSort.java
<strong> |
</strong> |
public class SelectionSort { |
public static void main(String[] args) { |
int array[] = new int [ 5 ]; |
for ( int index= 0 ; index<array.length; index++) { |
array[index] = ( int ) (Math.random()* 100 ); |
} |
for ( int index= 0 ; index<array.length; index++) { |
System.out.print(array[index] + ” “); |
} |
System.out.println(); |
18 |
19 | int x, y, min; |
20 | for (x= 0 ; x<array.length; x++) { |
21 | min = array[x]; |
22 | for (y=x+ 1 ; y<array.length; y++) { |
23 | if (array[y] < array[x]) { |
24 | min = array[y]; |
25 | int temp = min; |
26 | array[y] = array[x]; |
27 | array[x] = temp; |
28 | } |
29 | } |
30 | } |
31 |
32 | for ( int index= 0 ; index<array.length; index++) { |
33 | System.out.print(array[index] + ” “); |
34 | } |
35 | System.out.println(); |
36 | } |
37 |
38 | } |
9 69 96 97 47
9 47 69 96 97
Nama program: InsertionSort.java
01 | public class InsertionSort { |
02 |
03 | public static void main(String[] args) { |
04 |
05 | int array[] = new int [ 5 ]; |
06 | array[ 0 ] = 7 ; |
07 | array[ 1 ] = 5 ; |
08 | array[ 2 ] = 3 ; |
09 | array[ 3 ] = 8 ; |
10 | array[ 4 ] = 2 ; |
11 |
12 | int a; |
13 | int b; |
14 |
15 | for ( int index= 0 ; index<array.length; index++) { |
16 | System.out.print(array[index] + ” “); |
17 | } |
18 | System.out.println(“”); |
19 |
20 | for (a= 1 ; a<array.length; a++) |
21 | { |
22 | int temp = array[a]; |
23 | b = a; |
24 | while (b> 0 && array[b- 1 ] >= temp) |
25 | { |
26 | array[b] = array[b- 1 ]; |
27 | –b; |
28 | } |
29 | array[b] = temp; |
30 | } |
31 |
32 | for ( int index= 0 ; index<array.length; index++) { |
33 | System.out.print(array[index] + ” “); |
34 | } |
35 | System.out.println(“”); |
36 | } |
37 | } |
7 5 3 8 2
2 3 5 7 8
SORTING – GUI
Nama program: SortingGui.java
01 | import javax.swing.*; |
02 | import java.awt.*; |
03 | import java.awt.event.ActionListener; |
04 | import java.awt.event.ActionEvent; |
05 |
06 | public class SortingGui { |
07 |
08 | JPanel panelTengah = new JPanel(); |
09 | JPanel panelKanan = new JPanel(); |
10 | JPanel panelBawah = new JPanel(); |
11 | JPanel panelSemua = new JPanel(); |
12 | JTextField[] fieldArray = new JTextField[ 10 ]; |
13 | JLabel[] label = new JLabel[ 10 ]; |
14 | JTextField sisipElemen = new JTextField( 5 ); |
15 | JButton buttonSisip = new JButton(“Sisip”); |
16 | JTextField hapusElemen = new JTextField( 5 ); |
17 | JButton buttonHapus = new JButton(“Hapus”); |
18 | JButton buttonLinearSort = new JButton(“Linear Sort”); |
19 | JButton buttonInsertionSort = new JButton(“Insertion Sort”); |
20 | JButton buttonBubbleSort = new JButton(“Bubble Sort”); |
21 |
22 | SortingGui() { |
23 | BorderLayout bl = new BorderLayout(); |
24 | panelSemua.setLayout(bl); |
25 |
26 | GridLayout gl = new GridLayout( 10 , 2 ); |
27 | panelTengah.setLayout(gl); |
28 | for ( int x = fieldArray.length- 1 ; x >= 0 ; x–) { |
29 | panelTengah.add(label[x] = new JLabel(Integer.toString(x))); |
30 | panelTengah.add(fieldArray[x] = new JTextField( 5 )); |
31 | } |
32 | panelSemua.add(“Center”, panelTengah); |
33 |
34 | GridLayout gl2 = new GridLayout( 2 , 2 ); |
35 | panelKanan.setLayout(gl2); |
36 | panelKanan.add(sisipElemen); |
37 | panelKanan.add(buttonSisip); |
38 | panelKanan.add(hapusElemen); |
39 | panelKanan.add(buttonHapus); |
40 | panelSemua.add(“North”, panelKanan); |
41 |
42 | GridLayout gl3 = new GridLayout( 2 , 2 ); |
43 | panelBawah.setLayout(gl3); |
44 | panelBawah.add(buttonLinearSort); |
45 | panelBawah.add(buttonInsertionSort); |
46 | panelBawah.add(buttonBubbleSort); |
47 | panelSemua.add(“South”, panelBawah); |
48 |
49 | JFrame jendela = new JFrame(“Sorting GUI”); |
50 | jendela.setContentPane(panelSemua); |
51 | //jendela.setSize(200,400); |
52 | //jendela.setResizable(false); |
53 | jendela.pack(); |
54 | jendela.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); |
55 | jendela.setVisible( true ); |
56 |
57 | ProsesSorting proses = new ProsesSorting( this ); |
58 | buttonSisip.addActionListener(proses); |
59 | buttonHapus.addActionListener(proses); |
60 | buttonLinearSort.addActionListener(proses); |
61 | buttonInsertionSort.addActionListener(proses); |
62 | buttonBubbleSort.addActionListener(proses); |
63 | } |
64 |
65 | public static void main(String[] args) { |
66 | SortingGui sort = new SortingGui(); |
67 | } |
68 | } |
001 | import java.awt.Color; |
002 | import javax.swing.JOptionPane; |
003 | import java.awt.event.*; |
004 | public class ProsesSorting implements ActionListener { |
005 |
006 | SortingGui induk; |
007 | int array[] = new int [ 10 ]; |
008 | int nElemen = 0 ; |
009 |
010 | ProsesSorting(SortingGui induk) { |
011 | this .induk = induk; |
012 | } |
013 |
014 | public void actionPerformed(ActionEvent kejadian) { |
015 |
016 | if (kejadian.getSource() == induk.buttonSisip) { |
017 | array[nElemen] = Integer.parseInt(induk.sisipElemen.getText()); |
018 | //int sisip = Integer.parseInt(induk.sisipElemen.getText()); |
019 | induk.fieldArray[nElemen].setText(Integer.toString(array[nElemen])); |
020 | induk.fieldArray[nElemen].setBackground(Color.green); |
021 | induk.sisipElemen.setText(“”); |
022 | nElemen++; |
023 | tampil(); |
024 | } |
025 | else if (kejadian.getSource() == induk.buttonHapus){ |
026 | int j; |
027 |
028 | for ( int x= 0 ; x<nElemen; x++) { |
029 | induk.fieldArray[x].setText(“”); |
030 | } |
031 |
032 | for (j= 0 ; j<nElemen; j++){ |
033 | if ( Integer.parseInt(induk.hapusElemen.getText()) == array[j] ) |
034 | break ; |
035 | } |
036 | if (j==nElemen) |
037 | JOptionPane.showMessageDialog( null , “tidak ada”); |
038 | else |
039 | { |
040 | for ( int k=j; k<nElemen; k++) { |
041 | array[k] = array[k+ 1 ]; |
042 | //nElemen–; |
043 | } |
044 | nElemen–; |
045 | induk.fieldArray[nElemen].setBackground(Color.white); |
046 | } |
047 | induk.hapusElemen.setText(“”); |
048 | tampil(); |
049 | } else if (kejadian.getSource() == induk.buttonLinearSort) { |
050 | for ( int x = 0 ; x<nElemen- 1 ; x++) { |
051 | for ( int y = x+ 1 ; y<nElemen; y++) { |
052 | if (array[x] > array[y]){ |
053 | int temp = array[x]; |
054 | array[x] = array[y]; |
055 | array[y] = temp; |
056 | } |
057 | } |
058 | } |
059 | tampil(); |
060 | } else if (kejadian.getSource() == induk.buttonInsertionSort) { |
061 | int in, out; |
062 | for (out= 1 ; out<nElemen; out++) |
063 | { |
064 | int temp = array[out]; |
065 | in = out; |
066 | while (in> 0 && array[in- 1 ] >= temp) |
067 | { |
068 | array[in] = array[in- 1 ]; |
069 | –in; |
070 | } |
071 | array[in] = temp; |
072 | } |
073 | tampil(); |
074 |
075 | } else if (kejadian.getSource() == induk.buttonBubbleSort) { |
076 | for ( int x = nElemen- 1 ; x > 1 ; x–) { |
077 | for ( int y = 0 ; y < x; y++) { |
078 | if (array[y] > array[y + 1 ]) { |
079 | int temp = array[y]; |
080 | array[y] = array[y + 1 ]; |
081 | array[y + 1 ] = temp; |
082 | } |
083 | } |
084 | } |
085 | tampil(); |
086 | } |
087 |
088 | } |
089 |
090 | public void tampil(){ |
091 | for ( int x= 0 ; x<nElemen; x++) { |
092 | induk.fieldArray[x].setText(” “); |
093 | } |
094 |
095 | for ( int x= 0 ; x<nElemen; x++) { |
096 | induk.fieldArray[x].setText(Integer.toString(array[x])); |
097 | } |
098 | } |
099 |
100 | } |
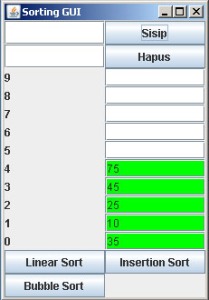
Elemen array sebelum diurutkan
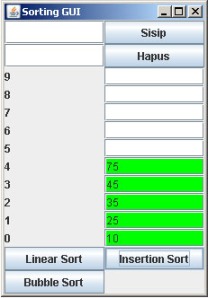
Elemen array setelah diurutkan
0 komentar:
Posting Komentar
Please Give Your Feedback Or Message.
Thank You!!?